Blog
How to Implement Machine Learning Algorithms in Python from Scratch
- June 23, 2023
- Posted by: Oyesh
- Category: Education Online Learning Guide Technology
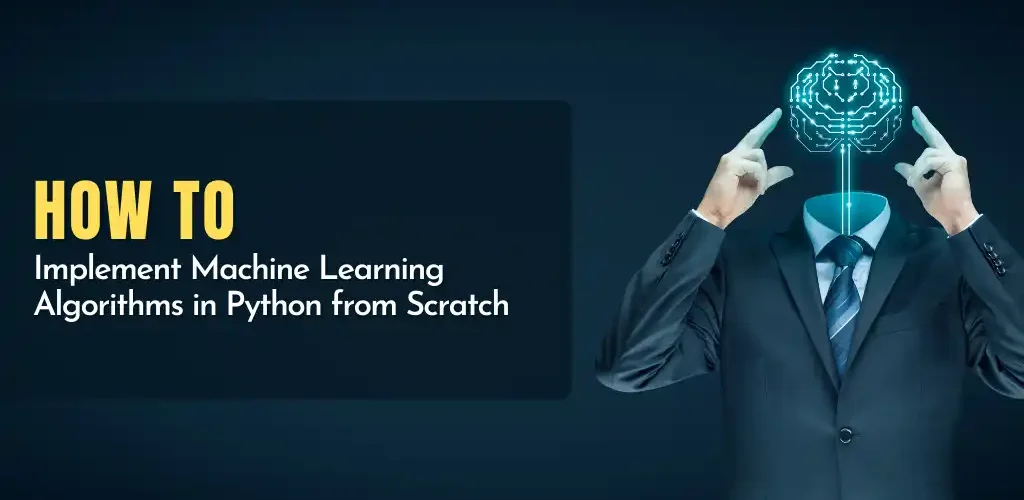
Introduction
As we navigate the digital age, machine learning has emerged as a vital technology, driving innovation across various sectors. With Python’s simplicity and extensive libraries, it has become the go-to language for implementing machine learning algorithms. In this blog post, we will delve into how you can implement machine learning algorithms in Python from scratch. Whether you’re a seasoned coder or a beginner in the field, you will find this guide helpful and insightful.
Understanding the Basics: What is Machine Learning?
Machine learning, a branch of artificial intelligence, allows computers to learn from data without being explicitly programmed. It uses algorithms that iteratively learn from the data, allowing the system to independently adapt to new scenarios. Machine learning algorithms in Python are utilized across many industries, aiding in decision making, predictive analysis, and pattern recognition.
Why Use Python for Machine Learning?
Python’s appeal lies in its simplicity, versatility, and extensive library support. It’s favored by developers and data scientists worldwide for its readability, making it perfect for beginners looking to implement machine learning algorithms. Python’s robust libraries, such as NumPy, Pandas, Matplotlib, and Scikit-learn, simplify the process of creating complex algorithms.
Getting Started with Python and Machine Learning
Before we delve into the process of implementing machine learning algorithms in Python from scratch, you’ll need to have Python installed on your system. Additionally, you should have a basic understanding of Python syntax, variables, loops, and functions.
Key Steps to Implementing Machine Learning Algorithms in Python
Implementing machine learning algorithms in Python involves a systematic process. Here are the key steps:
Step 1: Importing Libraries
Start by importing the necessary Python libraries. The most commonly used libraries for machine learning are NumPy, Pandas, Matplotlib, and Scikit-learn.
Step 2: Data Preprocessing
Data preprocessing is a crucial step in machine learning. This stage involves cleaning and transforming raw data into a format that the machine learning model can understand.
Step 3: Selecting a Machine Learning Algorithm
There are various types of machine learning algorithms in Python, each suitable for different kinds of problems. The choice of algorithm depends on the nature of the data and the problem you’re trying to solve.
Step 4: Training the Algorithm
Once you’ve selected an algorithm, the next step is to train it. This involves providing the algorithm with training data, from which it learns patterns and information.
Step 5: Testing the Algorithm
After training the algorithm, you need to test it using testing data. This stage is crucial for assessing the model’s performance.
Step 6: Evaluation and Optimization
Finally, evaluate your machine learning model using appropriate metrics, and optimize the algorithm for better performance if necessary.
Implementing Machine Learning Algorithms in Python: A Detailed Walkthrough
Having understood the key steps involved, let’s now delve into a detailed guide on how to implement two commonly used machine learning algorithms in Python from scratch: linear regression and k-nearest neighbors.
Linear Regression in Python
Linear regression is a popular algorithm in machine learning for predicting a continuous outcome variable (Y) based on one or more predictor variables (X).
Here’s how to implement it from scratch:
- Import Necessary Libraries: As always, we begin by importing the necessary libraries.
- Generate or Load the Dataset: You can either generate a dataset or load one for regression analysis.
- Implement the Algorithm: Implement the linear regression algorithm, which involves calculating the slope and intercept of the best fit line.
- Train the Model: Split the data into a training set and a testing set. Use the training set to train the model.
- Test the Model: Test the model using the testing set and evaluate its performance.
K-Nearest Neighbors (K-NN) in Python
K-NN is a simple, easy-to-implement supervised machine learning algorithm that can be used for both classification and regression.
Here’s how to implement it from scratch:
- Import Necessary Libraries: Begin by importing the necessary Python libraries.
- Generate or Load the Dataset: Generate or load a dataset for classification or regression.
- Implement the Algorithm: Implement the K-NN algorithm, which involves finding the K nearest neighbors and using majority vote or averaging for prediction.
- Train the Model: Split the data into a training set and a testing set, and then use the training set to train the model.
- Test the Model: Finally, test the model using the testing set and evaluate its performance.
Leveraging Python’s Powerful Libraries
While implementing machine learning algorithms in Python from scratch is a fantastic way to learn and understand them, in a real-world setting, you’ll often leverage powerful libraries such as Scikit-learn. These libraries simplify the process, making it easier to implement complex machine learning algorithms.
Wrapping Up
Implementing machine learning algorithms in Python from scratch might seem daunting, but with practice and persistence, it becomes an achievable task. It offers you a deep understanding of how the algorithms work and allows you to customize them to suit your specific needs.
As we approach the conclusion of this article, it’s essential to note that understanding and implementing machine learning algorithms is just the beginning. If you want to delve deeper and explore advanced concepts in data science, consider enrolling in our Advanced Data Science course.
Mastering the implementation of machine learning algorithms in Python is a valuable skill, opening a world of opportunities in the tech industry. So, roll up your sleeves, get your Python environment set up, and start coding! Your machine learning journey is just beginning.
Frequently Asked Questions
Q: What are the prerequisites for implementing machine learning algorithms in Python from scratch?
A: You should have a basic understanding of Python programming, including knowledge of variables, loops, and functions. Familiarity with libraries like NumPy, Pandas, and Matplotlib can be beneficial. A basic understanding of machine learning concepts and algorithms is also necessary.
Q: Why is Python a popular choice for implementing machine learning algorithms?
A: Python is known for its simplicity, readability, and extensive libraries. These characteristics make it perfect for implementing machine learning algorithms. Libraries such as NumPy, Pandas, and Scikit-learn provide powerful tools for data manipulation and analysis, which are crucial in machine learning.
Q: What are some of the most commonly used machine learning algorithms in Python?
A: Some of the most commonly used machine learning algorithms include linear regression, logistic regression, decision trees, random forest, K-nearest neighbors (K-NN), and support vector machines (SVM). The choice of algorithm depends on the problem at hand.
Q: Can I implement machine learning algorithms in Python without using any libraries?
A: Yes, it’s possible to implement machine learning algorithms from scratch without using any specific machine learning libraries. However, this is more complex and time-consuming. Python libraries like Scikit-learn simplify the process of implementing and working with machine learning algorithms.
Q: What’s the importance of data preprocessing in implementing machine learning algorithms in Python?
A: Data preprocessing is a crucial step in machine learning. It involves cleaning the data (handling missing values, removing outliers) and transforming it (normalization, encoding categorical variables) to a format that the machine learning algorithm can work with. Without proper preprocessing, the performance of your machine learning model may be negatively impacted.